25-12-2022 - Sparkfun Redboard Artemis Serial Out.
Missie:
Met een Arduino(achtige) gaan we een toestelletje bouwen om via de UART een ASCII telegram te versturen.
Later kunnen we dit mechanisme gebruiken als test instrument.
In het verloop van mijn avontuur ben ik ar achter gekomen dat een Arduino Uno toch te weining geheugen heeft om de opdracht te vervullen.
Ook is het handig een echte UART te gerbuiken om het aantal data-bits, stop-bits en pariteit te kunnen beinvloeden.
Dit geeft een veel flexibeler eindresultaat
De stap van Uno naar Mega was mij te groot vanwege de footprint van de Mega.
Ik ben uitgekomen bij een Sparkfun Redboard Artemis.
Op bovenstaande pagina zijn links naar de beschrijving, de features en de documentatie te vinden.
Sparkfun Redboard Artemis in Arduino IDE.
Op de Artemis gaan we een SD kaart shield plaatsen zoals rechts in de kolom te zien.
Op de SD kaart wordt het seriele telegram gezet zoals in onderstaande samenvatting te zien.
Informatie over het SD kaart shield vind je hier..
En er is ook een nog goedkopere oplossing..
Maar allereerst gaan we de situatie zoals we die hadden in de vorige blog, herstellen op de Artemis.
We gebruiken nu de SerialSoftware bibliotheek niet maar gaan de tweede UART gebruiken. Volgens het schema zit die op de pinnen 1 (RX1) en 2 (TX1). Tevens te zien op het bordje.
De pinnen van UART0, die gebruikt wordt voor de bootloader en voor de terminal, zitten op de achterkant van het bord. De pinnen RX0 en TX0.
LET OP: De Artemis gebruikt een 3.3 Volt signaal niveau.
Heb je een 5 Volt signaal niveau nodig dan moet je er een level shifter zoals bijvoorbeeld deze gebruiken.
Voor het overzicht heb ik het setup gedeelte in een apart bestand gezet: setup.h.
Hier beneden volgen de listings, eerst de settings, dan de setup en vervolgens het main gedeelte.
settings.h
//----------------------------------------------------------------------------+
// File : settings.h |
// Autor : Wim Cranen |
// Website : https://www.wccandm.nl |
// Date design : 5 december 2022 |
// Date tested : |
// Version : 0.1 - initial release |
// : 0.2 - variable changes |
// : 0.3 - changed program |
// : 0.4 - reshuffeld program |
// : 0.5 - setup routines to setup.h |
// Test result : v0.5 is OK |
//----------------------------------------------------------------------------+
// Revision history : |
// v0.2 - changed variables according to Barrgroup recommendations |
// v0.3 - changed program for Sparkfun Redboard Artemis |
// v0.4 - reshuffeld the setup and teh loop in bits and peices |
// v0.5 - moved the setup routines to a file called setup.h |
//----------------------------------------------------------------------------+
// --- Defines for SerialOut.ino ----------------------------------------------
#define BAUDRATE0 9600 // Baudrate for the terminal program
#define CONFIG0 SERIAL_8N1 // Configuration for the terminal program
#define P1VERSION 2 // Define the version for P1 port
// -- Get the version and prepare for the correct RS232 parameters ------------
#if P1VERSION == 2
#define BAUDRATE1 9600 // Baud rate for the UART1 using v2
#define CONFIG1 SERIAL_7E1 // Configuration for the UART1 using v2
#else
#define BAUDRATE2 115200 // Baud rate for the UART1 using v4, v5
#define CONFIG1 SERIAL_8N1 // Configuration for the UART1 using v4, v5
#endif
#define SWVERSION "v0.4" // Version number
// constants won't change:
const uint32_t BLINKINT = 100; // interval at which to blink (milliseconds)
const uint32_t SENDINT = 5000; // interval at which to send (milliseconds)
const uint16_t intLedPin = LED_BUILTIN; // the number of the LED pin
// Variables will change:
uint16_t ledState = LOW; // ledState used to set the LED
uint32_t prevBlinkMillis = 0; // will store last time LED was updated
uint32_t prevSendMillis = 0; // will store last time text was send
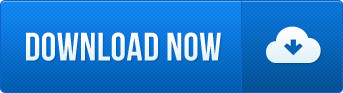
In de definitie wordt een variabele P1VERSION gebruikt. Als deze niet op 2 staat worden de parameters voor de P1 poort automatisch aangepast. Zie de tabel in het artikel over de P1 monitor (komt later).
setup.h
//----------------------------------------------------------------------------+
// File : setup.h |
// Autor : Wim Cranen |
// Website : https://www.wccandm.nl |
// Date design : 5 december 2022 |
// Date tested : |
// Version : 0.1 - initial release |
// : 0.2 - variable changes |
// : 0.3 - changed program |
// : 0.4 - reshuffeld program |
// : 0.5 - setup routines to setup.h |
// Test result : v0.5 is OK |
//----------------------------------------------------------------------------+
// Revision history : |
// v0.2 - changed variables according to Barrgroup recommendations |
// v0.3 - changed program for Sparkfun Redboard Artemis |
// v0.4 - reshuffeld the setup and teh loop in bits and peices |
// v0.5 - moved the setup routines to a file called setup.h |
//----------------------------------------------------------------------------+
//--- routines called in setup() ----------------------------------------------
void setupIntLedPin() // --- set internal ledpin for output -------------------
{
Serial.println("Setup: +--- Setting internal LED for OUTPUT");
pinMode(intLedPin, OUTPUT);
} //setupIntLedPin()
void setupSerial0() // --- // start serial terminal (UART0) -------------------
{
Serial.begin(BAUDRATE0, CONFIG0);
while (!Serial)
{ // wait for serial-0 ready
delay(100);
}
Serial.println("Setup: +--- Terminal window started (UART0).");
Serial.print("Setup: +--- Software version ");
Serial.println(SWVERSION);
} // setupSerial0()
void setupSerial1() // --- start serial1 UART1 -------------------------------
{
Serial1.begin(BAUDRATE1, CONFIG1);
while (!Serial1)
{ // wait for serial-1 ready
delay(100);
Serial.println("Waiting for UART1 to start.");
}
Serial.println("Setup: +--- Serial1 IO started (UART1).");
} // setupSerial1()
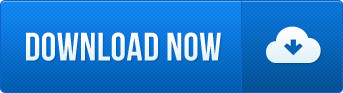
SerialOut.ino
//----------------------------------------------------------------------------+
// Program : SerialOut.ino |
// Autor : Wim Cranen |
// Website : https://www.wccandm.nl |
// Date design : 20 december 2022 |
// Date tested : xx december 2022 |
// Version : 0.1 - initial release |
// : 0.2 - variable changes |
// : 0.3 - changed program |
// : 0.4 - reshuffeld program |
// : 0.5 - setup routines to setup.h |
// Test result : v0.5 is OK |
//----------------------------------------------------------------------------+
// Revision history : |
// v0.2 - changed variables according to Barrgroup recommendations |
// v0.3 - changed program for Sparkfun Redboard Artemis |
// v0.4 - reshuffeld the setup and teh loop in bits and peices |
// v0.5 - moved the setup routines to a file called setup.h |
//----------------------------------------------------------------------------+
//--- Include libraries -------------------------------------------------------
#include <arduino.h>
//--- Settings in local directory ---------------------------------------------
#include "settings.h" // file for settings, variables and constants
#include "setup.h" // setup routines
void setup() // --- setup() main routine --------------------------------------
{
setupIntLedPin(); // setup internal LED pin for output
setupSerial0(); // initialize and start UART0
setupSerial1(); // initialize and start UART1
} // void setup()
//--- void blink() blink the internal LED routine -----------------------------
void blink()
{
//--- blinking the LED -------------------------------------------------------
// This is just to see that the program is running
// check to see if it's time to blink the LED; that is, if the difference
// between the current time and last time you blinked the LED is bigger than
// the interval at which you want to blink the LED.
uint32_t curBlinkMillis = millis();
// check if blink time elapsed
if (curBlinkMillis - prevBlinkMillis >= BLINKINT)
{
// save the last time you blinked the LED
prevBlinkMillis = curBlinkMillis;
// if the LED is off turn it on and vice-versa:
ledState = !(digitalRead(intLedPin));
digitalWrite(intLedPin, ledState);
} // if blink millis elapsed
} // void blink()
//--- void sendText() sends the text to the serial output ---------------------
void sendText()
{
//--- sending the text ------------------------------------------------------
uint32_t curSendMillis = millis();
// check if send time elapsed
if (curSendMillis - prevSendMillis >= SENDINT)
{
// control LED on
digitalWrite(intLedPin, HIGH);
// save the last time you send the text
prevSendMillis = curSendMillis;
// print to the terminal windos
Serial.println("Hello to the UART0 world");
Serial1.println("Hello to the UART1 world");
// control LED off
digitalWrite(intLedPin, LOW);
} // if send millis elapsed
} // void sendText()
//--- loop() is main() --------------------------------------------------------
void loop()
{
sendText();
//blink();
} // void loop()
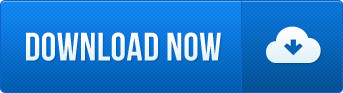
Zoals je kunt zien is de blink() routine in een commentaar regel is opgenomen.
Tevens zijn in de sendText() routine twee regels opgenomen om de LED bij het begin van de routine aan te zetten en bij het eide van de routine weer uit te zetten.
Dit ter controle van de functie.
Daarna verbinden we een RS232-FTDI kabeltje aan de pinnen 1 en 2, en zie hier beneden het resultaat in CoolTerm.
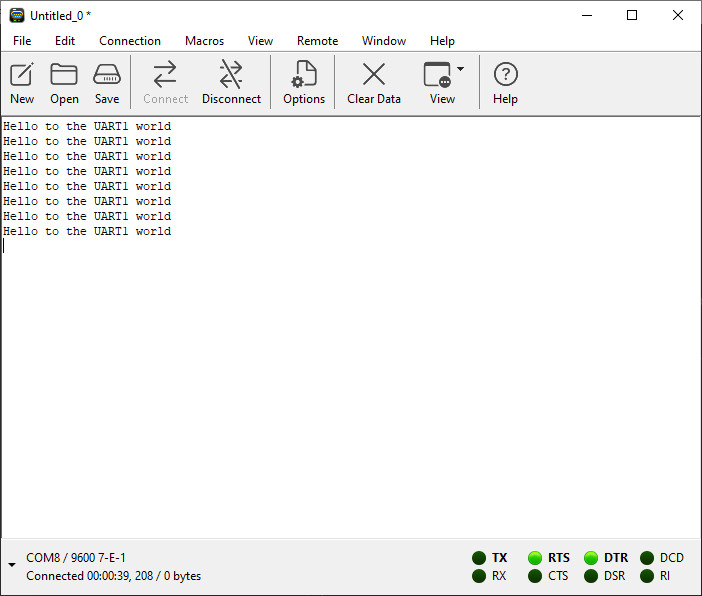
Je ziet dat CoolTerm (en ook de UART1) prima reageren op de instellingen van de UART.
Hierna gaan we de zaak wat gecompliceerder maken. Zoals in het begin beloofd, gaan we een bestand op een SD kaart zetten, dat bestand lezen,
het vervolgens via de UART1 naar buiten sturen en controleren met CoolTerm.
Voor de informatie m.b.t. de SD kaart een verwijzing naar de bibliotheken.
Voor de informatie m.b.t. de toepassingen van een SD kaart, verwijs ik naar deze voorbeelden
en naar de voorbeelden in de IDE, maar ook naar het voorbeeld programma van de betreffende SD kaart (zie boven).
Op de SD kaart gaan we het volgende tekst bestand zetten, en ja dat is het telegram van een "slimme" meter, versie 5, met CRC.
TELEGRAM.TXT
/FLU5\253769484_A
0-0:96.1.4(50215)
0-0:96.1.1()
0-0:1.0.0(210410103019S)
1-0:1.8.1(001869.223*kWh)
1-0:1.8.2(002598.088*kWh)
1-0:2.8.1(000535.014*kWh)
1-0:2.8.2(000175.049*kWh)
0-0:96.14.0(0002)
1-0:1.7.0(00.052*kW)
1-0:2.7.0(00.000*kW)
1-0:21.7.0(00.000*kW)
1-0:41.7.0(00.000*kW)
1-0:61.7.0(00.081*kW)
1-0:22.7.0(00.004*kW)
1-0:42.7.0(00.023*kW)
1-0:62.7.0(00.000*kW)
1-0:32.7.0(237.8*V)
1-0:52.7.0(238.1*V)
1-0:72.7.0(241.1*V)
1-0:31.7.0(000.74*A)
1-0:51.7.0(000.52*A)
1-0:71.7.0(000.69*A)
0-0:96.3.10(1)
0-0:17.0.0(999.9*kW)
1-0:31.4.0(999*A)
0-0:96.13.0()
0-1:24.1.0(003)
0-1:96.1.1()
0-1:24.4.0(1)
0-1:24.2.3(210410102502S)(00012.445*m3)
!1CCE
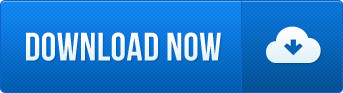
Kopieer dit bestand naar de SD kaart en steek de SD kaart in het SD kaart lezer shield.
In settings.h moeten we enkele aanpassingen maken.
settings.h
//----------------------------------------------------------------------------+
// File : settings.h |
// Autor : Wim Cranen |
// Website : https://www.wccandm.nl |
// Date design : 5 december 2022 |
// Date tested : |
// Version : 0.1 - initial release |
// : 0.2 - variable changes |
// : 0.3 - changed program |
// : 0.4 - reshuffeld program |
// : 0.5 - setup routines to setup.h |
// : 0.6 -- basic SD card functions |
// Test result : v0.6 is OK |
//----------------------------------------------------------------------------+
// Revision history : |
// v0.2 - changed variables according to Barrgroup recommendations |
// v0.3 - changed program for Sparkfun Redboard Artemis |
// v0.4 - reshuffeld the setup and the loop in bits and pieces |
// v0.5 - moved the setup routines to a file called setup.h |
// v0.6 - added SD card basic functionality |
//----------------------------------------------------------------------------+
// --- Defines for SerialOut.ino ----------------------------------------------
#define BAUDRATE0 9600 // Baudrate for the terminal program
#define CONFIG0 SERIAL_8N1 // Configuration for the terminal program
#define FILENAME "TELEGRAM.TXT" // Filename for the file on SD card
// -- Get the version and prepare for the correct RS232 parameters ------------
#define P1VERSION 2 // Define the version for P1 port
#if P1VERSION == 2
#define BAUDRATE1 9600 // Baud rate for the UART1 using v2
#define CONFIG1 SERIAL_7E1 // Configuration for the UART1 using v2
#else
#define BAUDRATE2 115200 // Baud rate for the UART1 using v4, v5
#define CONFIG1 SERIAL_8N1 // Configuration for the UART1 using v4, v5
#endif
#define SWVERSION "v0.6" // Version number
// --- Constants won't change -------------------------------------------------
const uint16_t BLINKINT = 100; // interval at which to blink (milliseconds)
const uint32_t SENDINT = 20000; // interval at which to send (milliseconds)
const uint16_t IntLedPin = LED_BUILTIN; // the number of the LED pin
const uint16_t CheckLEDPin = 2; // define the LED pin for the check signal
const uint16_t ChipSelect = 10; // define the chip select pin for SD card
// --- Variables will change --------------------------------------------------
uint16_t ledState = LOW; // ledState used to set the LED
uint32_t prevBlinkMillis = 0; // will store last time LED was updated
uint32_t prevSendMillis = 0; // will store last time text was send
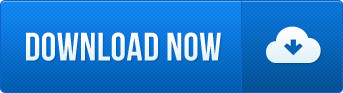
Dan het setup.h gedeelte aanpassen voor de extra functionaliteit rondom de SD kaart.
setup.h
//----------------------------------------------------------------------------+
// File : setup.h |
// Autor : Wim Cranen |
// Website : https://www.wccandm.nl |
// Date design : 5 december 2022 |
// Date tested : |
// Version : 0.1 - initial release |
// : 0.2 - variable changes |
// : 0.3 - changed program |
// : 0.4 - reshuffeld program |
// : 0.5 - setup routines to setup.h |
// : 0.6 -- basic SD card functions |
// Test result : v0.6 is OK |
//----------------------------------------------------------------------------+
// Revision history : |
// v0.2 - changed variables according to Barrgroup recommendations |
// v0.3 - changed program for Sparkfun Redboard Artemis |
// v0.4 - reshuffeld the setup and teh loop in bits and peices |
// v0.5 - moved the setup routines to a file called setup.h |
// v0.6 - added SD card basic functionality |
//----------------------------------------------------------------------------+
// === set up variables using the SD utility library functions ================
// The circuit:
// SD card attached to SPI bus as follows:
// MOSI - pin 11
// MISO - pin 12
// CLK - pin 13
// CS - pin 10
Sd2Card mySDcard;
SdVolume mySDvolume;
SdFile mySDroot;
// === set up variable for the file ===========================================
File mySDfile;
// === routines called in setup() =============================================
// --- print Long Long number - be aware it is slow slow slow -----------------
void printLLNumber(uint64_t n, uint8_t base) {
unsigned char buf[16 * sizeof(long)];
unsigned int i = 0;
if (n == 0) {
Serial.print((char)'0');
return;
}
while (n > 0) {
buf[i++] = n % base;
n /= base;
}
for (; i > 0; i--)
Serial.print((char) (buf[i - 1] < 10 ?
'0' + buf[i - 1] :
'A' + buf[i - 1] - 10));
} // void printLLNumber(uint64_t n, uint8_t base)
// --- set internal ledpin for output -----------------------------------------
void setupIntLedPin() {
Serial.println("Setup: +--- Setting internal LED for OUTPUT");
pinMode(IntLedPin, OUTPUT);
} //setupIntLedPin()
// --- set check ledpin for output --------------------------------------------
void setupCheckLedPin() {
Serial.println("Setup: +--- Setting check LED for OUTPUT");
pinMode(CheckLEDPin, OUTPUT);
} //setupCheckLedPin()
// --- set internal chipselect for output -------------------------------------
void setupCSPin() {
Serial.println("Setup: +--- Setting CS pin for OUTPUT");
pinMode(ChipSelect, OUTPUT);
} //setupCSPin()
// --- start serial terminal (UART0) ------------------------------------------
void setupSerial0() {
Serial.begin(BAUDRATE0, CONFIG0);
while (!Serial) { // wait for serial-0 ready
delay(100);
}
Serial.println("Setup: +--- Terminal window started (UART0).");
Serial.print("Setup: +--- Software version ");
Serial.println(SWVERSION);
} // setupSerial0()
// --- start serial1 UART1 ----------------------------------------------------
void setupSerial1() {
Serial1.begin(BAUDRATE1, CONFIG1);
while (!Serial1) { // wait for serial-1 ready
delay(100);
Serial.println("Waiting for UART1 to start.");
}
Serial.println("Setup: +--- Serial1 IO started (UART1).");
} // setupSerial1()
// --- start SD card ----------------------------------------------------------
void SDcardInfo(){
Serial.println("Setup: +--- Initializing SDcard on CS 10");
setupCSPin();
// we'll use the initialization code from the utility libraries
// since we're just testing if the mySDcard is working!
if (!mySDcard.init(SPI_HALF_SPEED, ChipSelect)) {
Serial.println("Setup: +--- initialization failed. Things to check:");
Serial.println(" * is a mySDcard is inserted?");
Serial.println(" * Is your wiring correct?");
Serial.println(" * did you change the ChipSelect pin to match your shield or module?");
return;
} else {
Serial.println("Setup: +--- Wiring is correct and a mySDcard is present.");
}
// print the type of mySDcard
Serial.print("Setup: +--- Card type: ");
switch (mySDcard.type()) {
case SD_CARD_TYPE_SD1:
Serial.println("SD1");
break;
case SD_CARD_TYPE_SD2:
Serial.println("SD2");
break;
case SD_CARD_TYPE_SDHC:
Serial.println("SDHC");
break;
default:
Serial.println("Unknown");
}
// try to open the 'mySDvolume'/'partition' - it should be FAT16 or FAT32
if (!mySDvolume.init(mySDcard)) {
Serial.println("Setup: +--- Could not find FAT16/FAT32 partition.");
Serial.println(" * Make sure you've formatted the mySDcard");
return;
}
// print the type and size of the first FAT-type mySDvolume
uint64_t volumesize64;
uint32_t volumesize32;
Serial.print("Setup: +--- Volume type is FAT");
Serial.println(mySDvolume.fatType(), DEC);
volumesize64 = mySDvolume.blocksPerCluster(); // clusters are collections of blocks
volumesize64 *= mySDvolume.clusterCount(); // we'll have a lot of clusters
volumesize64 *= 512; // SD card blocks are always 512 bytes
Serial.print("Setup: +--- SDvolume size (bytes) : ");
printLLNumber(volumesize64, DEC);Serial.println();
Serial.print("Setup: +--- SDvolume size (Kbytes) : ");
volumesize32 = volumesize64/1024;
Serial.println(volumesize32);
Serial.print("Setup: +--- SDvolume size (Mbytes) : ");
volumesize32 /= 1024;
Serial.println(volumesize32);
Serial.println("Setup: +--- Files found on the mySDcard (name, date and size in bytes): ");
mySDroot.openRoot(mySDvolume);
// list all files in the mySDcard with date and size
mySDroot.ls(LS_R | LS_DATE | LS_SIZE);
} // SDcardInfo()
// --- start SD card ----------------------------------------------------------
void setupSDcard() {
Serial.println("Setup: +--- Initializing SD card...");
// see if the card is present and can be initialized:
if (!SD.begin(ChipSelect)) {
Serial.println("Setup: +--- ERROR: Card failed, or not present, program stopped!!");
// don't do anything more:
while (1);
}
Serial.println("Setup: +--- SDcard initialized.");
} // void setupSDcard()
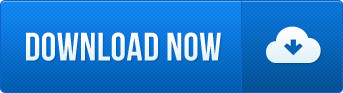
En tot slot het bestand SerialOut.ino aanpassen.
SerialOut.ino
//----------------------------------------------------------------------------+
// Program : SerialOut.ino |
// Autor : Wim Cranen |
// Website : https://www.wccandm.nl |
// Date design : 20 december 2022 |
// Date tested : xx december 2022 |
// Version : 0.1 - initial release |
// : 0.2 - variable changes |
// : 0.3 - changed program |
// : 0.4 - reshuffeld program |
// : 0.5 - setup routines to setup.h |
// : 0.6 -- basic SD card functions |
// Test result : v0.6 is OK |
//----------------------------------------------------------------------------+
// Revision history : |
// v0.2 - changed variables according to Barrgroup recommendations |
// v0.3 - changed program for Sparkfun Redboard Artemis |
// v0.4 - reshuffeld the setup and teh loop in bits and peices |
// v0.5 - moved the setup routines to a file called setup.h |
// v0.6 - added SD card basic functionality |
//----------------------------------------------------------------------------+
//--- Include libraries -------------------------------------------------------
#include <arduino.h> // only necessary for Visual Studio Code
#include <SPI.h> // SPI library
#include <SD.h> // SD card library
//--- Settings in local directory ---------------------------------------------
#include "settings.h" // file for settings, variables and constants
#include "setup.h" // setup routines
// --- setup() main routine ---------------------------------------------------
void setup(){
setupIntLedPin(); // setup internal LED pin for output
setupCheckLedPin(); // setup check LED pin for output
setupSerial0(); // initialize and start UART0
setupSerial1(); // initialize and start UART1
setupSDcard(); // initialize and start SD card
SDcardInfo(); // get info on the SD card
} // void setup()
//--- void blink() blink the internal LED routine -----------------------------
void blink(uint16_t pinNr, uint16_t interval) {
//--- blinking the LED ------------------------------------------------------
// This is just to see that the program is running
// check to see if it's time to blink the LED; that is, if the difference
// between the current time and last time you blinked the LED is bigger than
// the interval at which you want to blink the LED.
uint32_t curBlinkMillis = millis();
// check if blink time elapsed
if (curBlinkMillis - prevBlinkMillis >= interval) {
// save the last time you blinked the LED
prevBlinkMillis = curBlinkMillis;
// if the LED is off turn it on and vice-versa:
ledState = !(digitalRead(pinNr));
digitalWrite(pinNr, ledState);
} // if blink millis elapsed
} // void blink()
// --- void readFile() reads the file from the SD card and display on ---------
// ------- terminal and serial port -------------------------------------------
void readFile() {
uint32_t curSendMillis = millis();
uint32_t CharCount = 0;
digitalWrite(CheckLEDPin, LOW);
// check if send time elapsed
if (curSendMillis - prevSendMillis >= SENDINT) {
Serial.print(curSendMillis);
Serial.print(" - ");
Serial.println("Millis elapsed");
// save the last time you send the text
prevSendMillis = curSendMillis;
Serial.print("Opening file: ");
Serial.print(FILENAME);
Serial.println(" for reading.");
mySDfile = SD.open(FILENAME, FILE_READ);
if (mySDfile.available()){
Serial.println("File found");
// check LED on
digitalWrite(CheckLEDPin, HIGH);
while (mySDfile.available()){
char ch = mySDfile.read();
CharCount++;
Serial.print(ch);
Serial1.print(ch);
} // while
Serial.print("\n\nThe file contains : ");
Serial.print(CharCount);
Serial.println(" characters.\n\n");
// check LED off
digitalWrite(CheckLEDPin, LOW);
} //if
else {
Serial.println("+++ ERROR: File not found.");
} // else
mySDfile.close();
} // if send millis elapsed
} // void readFile()
//--- loop() is main() --------------------------------------------------------
void loop() {
readFile();
blink(IntLedPin, 500);
} // void loop()
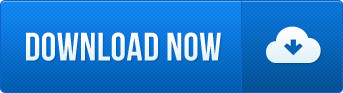
Compileer het geheel en stuur het naar de Sparkfun Redboard Artemis.
Daarna verbinden we een RS232-FTDI kabeltje aan de pinnen 1 en 2, en zie hier beneden het resultaat in CoolTerm.
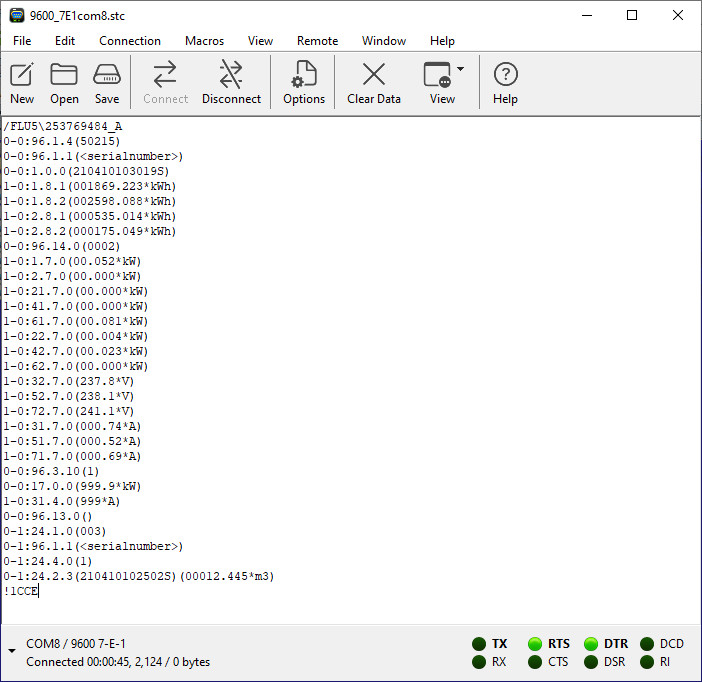
Je ziet dat CoolTerm (en ook de UART1) prima reageren op de instellingen van de UART.
Het telegram wordt prima weergegeven.
We hebben nu een omgeving gecreëeerd waarmee we een "slimme" meter kunnen simuleren.
Nu kunnen we ons concentreren op de volgende stap: het P1 telegram binnen lezen en decoderen.
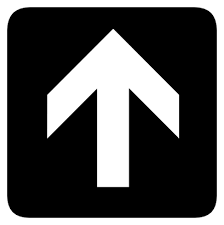